Many HTML Elements can be used as containers. That is, they can include other elements within them. The DIV is the most basic form of container in HTML, and most sheets will have many div blocks. For example, here’s a div block containing a heading and another div block containing a bunch of stats:
<div>
<h2>Stats</h2>
<div>
<span>Strength</span>
<input type="number" name="attr_strength" value="10" title="@{strength}">
<span>Dexterity</span>
<input type="number" name="attr_dexterity" value="10" title="@{dexterity}">
<span>Wisdom</span>
<input type="number" name="attr_wisdom" value="10" title="@{wisdom}">
</div>
</div>
Code language: HTML, XML (xml)
Here’s what that looks like since I used spans:
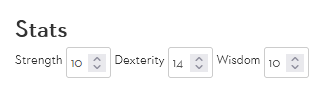
If you wanted to split the stats over multiple lines, you could add linebreaks, or add each line inside a container, like this:
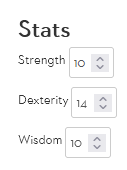
<div>
<h2>Stats</h2>
<div>
<p>
<span>Strength</span>
<input type="number" name="attr_strength" value="10" title="@{strength}">
</p>
<p>
<span>Dexterity</span>
<input type="number" name="attr_dexterity" value="10" title="@{dexterity}">
</p>
<p>
<span>Wisdom</span>
<input type="number" name="attr_wisdom" value="10" title="@{wisdom}">
</p>
</div>
</div>
Code language: HTML, XML (xml)
That would look like the picture to the right. Notice how the columns are not lined up well – you’ll find out how to solve that problem in CSS. The important thing to learn here is how to use containers.
Use indentation to show what is a container and what is contained. It’s not required – HTML will work if everything is typed in one line. HTML doesn’t care about line breaks and indentation.
But writing code like this makes things much more readable for humans looking at it, so you should always write code like this when you can. If you use a Code Editor, the program will have tools to organise code more easily.
Containers in HTML
The main reason to use containers is to group things together. As shown above, grouping everything inside a paragraph keeps those items together. DIV blocks are used to group things logically. You’ll find other advantages to grouping things sensibly when we look at CSS but for now it’s mostly for this kind of organisation.
Sections
The section tag works exactly like a div element and is basically identical in most ways. You could easily make your sheets without ever using a section element. The reason to use it is if there is some meaning to the group it contains. For example, you might use a section to contain everything related to a character description, or all a character’s saves, or spells.
DIV is a neutral tag – it doesn’t suggest that the things being contained have any special relationship to each other, whereas section does.
It’s a subtle distinction, explained better on other sites. One way to think about it: if it has its own heading, it might be a section. If there’s no heading, it probably isn’t a section.
As I said above, you can make a sheet without ever using a section tag. So if you feel you understand them, use them. If not, it’ll be fine if you don’t use them.
Details and Summary
Details is a very special container. You can have a summary, which you click to show increased detail. (See why the element has the name it does). For example, you might have a spell and show the name, and hide the full details of the spell until clicked.
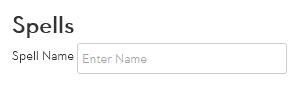
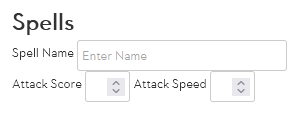
The image to the left is the normal view, but when you click the spell name, you get the image to the right. The code for that looks like this:
<h2>Spells</h2>
<details>
<span>Attack Score</span>
<input type="number" name="attr_spell_score">
<span>Attack Speed</span>
<input type="number" name="attr_spell_speed">
<summary>
<span>Spell Name</span>
<input type="text" name="attr_spell_name" placeholder="Enter Name">
</summary>
</details>
Code language: HTML, XML (xml)
Notice that the summary is always shown, and you put that in its own block. Everything else is only shown when you click the span.
You might put everything not in the summary in its own div block for easy readability, like so:
<details>
<div>
<span>Attack Score</span>
<input type="number" name="attr_spell_score">
<span>Attack Speed</span>
<input type="number" name="attr_spell_speed">
</div>
<summary>
<span>Spell Name</span>
<input type="text" name="attr_spell_name" placeholder="Enter Name">
</summary>
</details>
Code language: HTML, XML (xml)
Whether you write this code with a div block or not it works the same – the div block is just there to make it easier to see the details separate from the summary block.
One thing to be careful of with details elements: you need at least one non-interactive element in the summary like a span, heading, paragraph, etc. If the summary only contains inputs, textareas, or similar interactive elements, there’s no way to click the block to reveal the details. Try it again with this details block:
<details>
<div>
<span>Attack Score</span>
<input type="number" name="attr_spell_score">
<span>Attack Speed</span>
<input type="number" name="attr_spell_speed">
</div>
<summary>
<input type="text" name="attr_spell_name" placeholder="Enter Name">
</summary>
</details>
Code language: HTML, XML (xml)
Summary
There you have it – containers are great tools for organising a sheet, and making the code more readable. Details are special containers used to create interactive areas of the character sheet where you can reveal extra details.
Containers become much more flexible and powerful when combined with CSS, and you’ll find out about that in the next chapter of the guide.