In the last post, we looked at how we can a group of buttons linked to a single sheet worker. In this post, we’ll go even simpler, and show how a single sheet worker can represent multiple rolls through a query.
How Many Dice?
Imagine you have a single button on the sheet that represents several d6 rolls.
<button type="action" name="act_d6">Roll d6's</button>
Code language: HTML, XML (xml)
Then create a sheet worker for that button, like this.
on("clicked:d6", () => {
const roll_string = `&{template:default} {{name=@{character_name}}} {{?{How Many Dice?|1|2|3|4|5|6}d6=[[?{How Many Dice?}d6]]}}`;
startRoll(roll_string, roll => {
finishRoll(roll.rollId);
});
});
Code language: JavaScript (javascript)
Here we have a button that asks the user how many d6 they want to roll, then shows their sum. Some systems ask you to roll a pool of dice, and take the single highest die.
on("clicked:d6", () => {
const roll_string = `&{template:default} {{name=@{character_name}}} {{?{How Many Dice?|1|2|3|4|5|6}d6=[[?{How Many Dice?}d6kh1]]}}`;
startRoll(roll_string, roll => {
finishRoll(roll.rollId);
});
});
Code language: JavaScript (javascript)
Stat Query
Let’s say you want to use the stats from the character sheet, but don’t want to create a button for each. Let’s say you have a single button.
<button type="action" name="act_stat">Roll 2d20 + Stat</button>
Code language: HTML, XML (xml)
Then in your roll string, you have a query that lets players choose which stat to use.
on("clicked:stat", () => {
const roll_string = `&{template:default} {{name=@{character_name}}} {{?{Which Stat?|STR,@{str}|DEX,@{dex}|CON,@{con}|INT,@{int}|WIS,@{wis}|CHA,@{cha}}=[[1d20+?{Which Stat?}]]}}`;
startRoll(roll_string, roll => {
finishRoll(roll.rollId);
});
});
Code language: JavaScript (javascript)
If you want this to be run from chat, you’ll need to include a way for Roll20 to associate the value with a character, like using the character name or selected. For example:
on("clicked:stat", () => {
const roll_string = `&{template:default} {{name=@{selected|character_name}}} {{?{Which Stat?|STR,@{selected|str}|DEX,@{selected|dex}|CON,@{selected|con}|INT,@{selected|int}|WIS,@{selected|wis}|CHA,@{selected|cha}}=[[1d20+?{Which Stat?}]]}}`;
startRoll(roll_string, roll => {
finishRoll(roll.rollId);
});
});
Code language: JavaScript (javascript)
Showing A Label
The code above doesnt easily allow a descriptive label, like the name of the roll or a label for the stat used. This can be done pretty easily in the previous post by using the name of the button. But here we have only one button, so we need to use a more complex roll string. We can often avoid HTML entity substitution (shudder):
on("clicked:stat", () => {
const roll_string = `&{template:default} {{name=?{Name Of Roll} }} {{?{Which Stat?
|STR,STR (@{selected|str})=[[1d20+@{selected|str}]]
|DEX,DEX (@{selected|dex})=[[1d20+@{selected|dex}]]
|CON,CON (@{selected|con})=[[1d20+@{selected|con}]]
|INT,INT (@{selected|int})=[[1d20+@{selected|int}]]
|WIS,WIS (@{selected|wis})=[[1d20+@{selected|wis}]]
|CHA,CHA (@{selected|cha})=[[1d20+@{selected|cha}]]} }}`;
startRoll(roll_string, roll => {
finishRoll(roll.rollId);
});
});
Code language: JavaScript (javascript)
Here we have a roll that prompts the player for a name of the roll, then to pick a stat – which is then used in a d20+stat roll. That output might look like this:
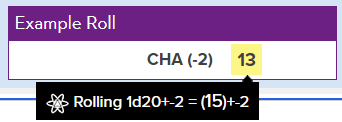
Conclusion
So there you have it. You can make simple rolls using queries. Any kind of valid roll expression can be used for the roll string. In the next posts, we’ll see how to do things you can’t do with roll20’s standard rolls.