The simple roll template described so far is for a specific roll macro. This one:
&{template:simple-roll} {{title=A Skill Roll}} {{roll=[[1d20+@{selected|skill}]]}}
Code language: Markdown (markdown)
You can vary this a little, but it alwyas expects {{title}}
and {{roll}}
to be there, whatever their values are. If you try to vary it, you’ll hit snags. Lets say you type this:
&{template:simple-roll} {{name=A Skill Roll}} {{My Skill=[[1d20+1]]}} {{Second=[[1d20+7]] }}
Code language: Markdown (markdown)

This is the result:
Let’s look back at the HTML for the rolltemplate.
<rolltemplate class="sheet-rolltemplate-simple-roll">
<div class="sheet-template-title">{{title}}</div>
<div class="sheet-roll-title">Roll</div>
<div class="sheet-roll-result">{{roll}}</div>
</rolltemplate>
Code language: HTML, XML (xml)
The rolltemplate call we used didn’t include title or roll, so there was nothing to show. The new keys, My Skill and Second, aren’t in the original code so they aren’t shown either. But the text Roll is hardcoded, so it was displayed even though there was nothing to show it with.
In this post, we’ll solve those problems.
Required Keys
One of the first things you need to do is identify which key-value pairs must always exist. It’s fine to have some required parts. If you create a rolltemplate specifically to handle attack rolls, you always want an attack value, for example.
The way the rolltemplate above is constructed, you must always have a title
and key-value pair for roll
. At least in theory. If your code doesn’t include any hard coded elements, those sections will be omitted. For example:

&{template:simple-roll} {{roll=[[1d20+1]]}}
Code language: Markdown (markdown)
If you look at the styling for that title, there is no defined height (line-height only counts if there is text, and there is no text). So you can get away with ommitting it here. It takes up no space.
But you can’t omit the roll. But what if we wanted to?
Optional Keys
This is where optional keys come in. There are keys that only show if they exisat when the template is called. You can do this:
<rolltemplate class="sheet-rolltemplate-simple-roll">
<div class="sheet-template-title">{{title}}</div>
{{#roll}}
<div class="sheet-roll-title">Roll</div>
<div class="sheet-roll-result">{{roll}}</div>
{{/roll}}
</rolltemplate>
Code language: HTML, XML (xml)
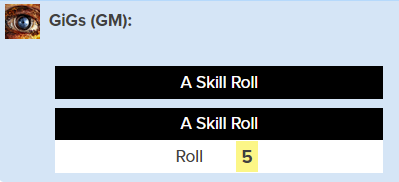
A block using an # before the key name says “show this only if the key exists, up to the /key“. Here are three examples. Directly below the Gigs (GM) name you see nothing – this is output omitting both the title and roll. It’s an empty line.
Following that is output using the title but no roll, and then title and roll.
You can see the roll has become optional. You could maybe use the title label to just enter text – a description. It would always have that black background which might not be ideal. So lets add an entry at the end just for description.
<rolltemplate class="sheet-rolltemplate-simple-roll">
<div class="sheet-template-title">{{title}}</div>
{{#roll}}
<div class="sheet-roll-title">Roll</div>
<div class="sheet-roll-result">{{roll}}</div>
{{/roll}}
{{#desc}}
<div class="sheet-desc">{{desc}}</div>
{{/desc}}
</rolltemplate>
Code language: HTML, XML (xml)
There’s a problem here though. We have used CSS grid styling to sort these into columns. Lets fix that by putting the grid only on the roll divs.
<rolltemplate class="sheet-rolltemplate-simple-roll">
<div class="sheet-template-title">{{title}}</div>
{{#roll}}
<duv class="sheet-roll">
<div class="sheet-roll-title">Roll</div>
<div class="sheet-roll-result">{{roll}}</div>
</div>
{{/roll}}
{{#desc}}
<div class="sheet-desc">{{desc}}</div>
{{/desc}}
</rolltemplate>
Code language: HTML, XML (xml)
We have two optional keys here. Now, we’d need to tweak the CSS.
.sheet-rolltemplate-simple-roll {
background-color: white;
line-height: 1.9em;
}
.sheet-rolltemplate-simple-roll .sheet-template-title {
background-color: black;
color: white;
text-align: center;
}
.sheet-rolltemplate-simple-roll span {
text-align: center;
}
.sheet-rolltemplate-simple-roll .sheet-roll {
display: grid;
grid-template-columns: 45% 45%;
column-gap: 10%;
}
.sheet-rolltemplate-simple-roll .sheet-roll div:nth-child(odd) {
text-align: right;
}
.sheet-rolltemplate-simple-roll .sheet-roll div:nth-child(even) {
text-align: left;
}
Code language: CSS (css)
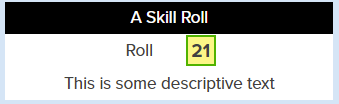
This works very similar to the old template. It sets everything to a white background, and the title to a black backgrouund. But now there is a sheet-roll class which has a CSS Grid assigned to it. The duvs under that one are set to left or right aligned, depending if they are even or odd.
You can see what that looks like with a title, roll, and desc all present. Any of those can be ommitted.
Technically speaking, the desc doesnt need to be optional, since it has no height. But this makes it more future proof for changes to the template. The same should be done to title – that can be left as an exercise to the reader.
All Keys – allProps
The current rolltemplate lets us declare some specific keys, and hide that part of the code if they aren’t available. But what if there are keys we didnt plan for. Like, say:
&{template:simple-roll} {{title=Several Rolls}} {{str=[[3d6kh3]]}} {{dex=[[3d6kh3]]}}
Code language: Markdown (markdown)
If we try this, the str and dex keys will be ignored, because we don’t have them built into the rolltemplate. Luckily, there’s a function that lets us handle unknown key-value pairs. That function is called allprops (which stands for All Properties).
allProps creates extra code for key-value pairs that havent been declared. For example, you can do this:
<rolltemplate class="sheet-rolltemplate-simple-roll">
{{#title}}<div class="sheet-template-title">{{title}}</div>{{/title}}
<div class="sheet-rolls">
{{#allprops()}}
<div class="sheet-roll-title">{{key}}</div>
<div class="sheet-roll-result">{{value}}</div>
{{/allprops()}}
</div>
{{#desc}}<div class="sheet-desc">{{desc}}</div>{{/desc}}
</rolltemplate>
Code language: HTML, XML (xml)
Notice how this is structured. The sheet-rolls class is placed outside allprops, so that all of its key-value pairs (here shown as {{key}} and {{value}}) are ordered into columns. The same css declarations can be used, only changing sheet-roll to sheet-rolls.
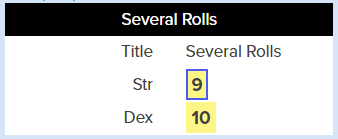
There is a problem though. Here’s what that roll looks like:
When you use allprops, it includes every key-value pair in the rolltemplate call, and we have title, str, and dex. We want to exclude title (and also desc if that is included).
Fortunately, the allprops function makes that easy. Just list the names of everything to want to exclude, on both the start (#) and ending (/) lines, like this:
<rolltemplate class="sheet-rolltemplate-simple-roll">
{{#title}}<div class="sheet-template-title">{{title}}</div>{{/title}}
<div class="sheet-rolls">
{{#allprops() title desc}}
<div class="sheet-roll-title">{{key}}</div>
<div class="sheet-roll-result">{{value}}</div>
{{/allprops() title desc}}
</div>
{{#desc}}<div class="sheet-desc">{{desc}}</div>{{/desc}}
</rolltemplate>
Code language: HTML, XML (xml)
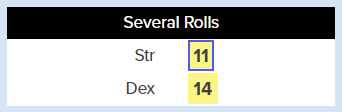
And now we have those excluded:
And there we go. We now have a rolltemplate we can use to make any number of rolls as long as we label them, and add a title and description element if we want to. Maybe we just include the descriptive element if we want to type some nicely formatted text.
In the next post, we’ll look at Conditional Keys – keys and values we do things with based on their value. This will get complicated, so buckle in.
Aside: The code examples above use divs for simplicity. Sometimes you’ll want to use <spans> or <p> or even <h2> and other headings then apply your own styling. The goal here is explaining rolltemplates and not CSS, so we have gone for a simple option.