- Anatomy of a Sheet Worker
- Events, and watching Attributes
- Variables – How to Name Things
- Arithmetic in Sheet Workers
- What If? in Sheet Workers
- JavaScript Objects
- Getting Loopy With JavaScript
- Logging in the Browser Console
- Strings, Arrays, and Loops
- Asynchronicity and Things to Avoid With Loops
- Changes and the eventInfo Object
- Action Buttons
- setAttrs and Saving Attributes
- Castle Falkenstein Design – Sheet Workers
- The Perils of Sheet Worker Functions
- The Script Block and Identifying Characters
- Arrays and Dropdowns
- Undefined and Other Error Values
- The Ternary Operator – The One-Line If
- Template Literals
- Functions and the Fat Arrow
- Strings in Sheet Workers
- A Sheet Worker Reprise
Like the last post, this isn’t an essential feature of JavaScript, but it’s so incredibly useful it is becoming standard. I speak the Template Literal, also often called the String Literal. It’s a newish way of handling Strings that adds incredibly useful features.
The Standard String
JavaScript has its own way of referencing and expanding strings.
const a_string = "This is a string.";
const string_part1 = "This is";
const string_part2 = "a string.";
const full_string = string_part1 + ' ' + string_part2;
Code language: JavaScript (javascript)
You can create strings and combine (concatenate) them using the + operator.
You can use two common types of quote – the ” and ‘ are interchangeable, and very handy for including quotes inside another quote:
const a_string = "She said, 'This is a string.'";
Code language: JavaScript (javascript)
The Third Type of Quote
You can use a ` (a backtick) as a quotation mark. They are usually found to the left of the number key on a standard keyboard.
const a_string = 'She said, `This is a string.`';
Code language: JavaScript (javascript)
I have seen people mistake them for normal quotes, but though they look similar, they are different. You can use them as normal quotation marks. But any text enclosed by them becomes a template literal, which creates some special benefits.
The Template Part of Template Literal
JavaScript, and HTML too, ignores spaces generally. This is not true in a template literal. Imagine you wanted to print something like this, spread across three lines:
on('sheet:opened', function() {
console.log("this is a test.
A Stat: a value
Another Stat: a value");
);
Code language: JavaScript (javascript)
You would have to try printing something like this.
on('sheet:opened', function() {
console.log("this is a test." +
" A Stat: a value" +
" Another Stat: a value");
);
Code language: JavaScript (javascript)
But that would be printed all along one line. To split into multiline, you’d need to insert the newline command:
on('sheet:opened', function() {
console.log("this is a test.\n" +
" A Stat: a value\n" +
" Another Stat: a value");
);
Code language: JavaScript (javascript)
That really isn’t very readable. But a template literal is much cleaner:
on('sheet:opened', function() {
console.log(`this is a test.
A Stat: a value
Another Stat: a value`);
);
Code language: JavaScript (javascript)
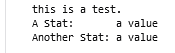
This respects spaces and lines exactly as you typed them. Everything inside the backticks (`) is printed exactly as you typed it, complete with spaces, lines, and indents.
This might sound like a little thing, but you’ll find many situations where it is very useful.
Including JS Inside a Template Literal
The other big feature of template literals, the killer app, is the way you can include code blocks inside a template literal using the ${ } operator. This can look ugly the first couple of times you use it but rapidly becomes second nature.
Let’s say you want to build a string that produces:
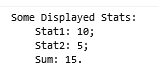
There are a number of ways you could build this output. A ‘common ‘simple’ one might be:
on('sheet:opened', function() {
getAttrs(['stat1', 'stat2'], function(values) {
const stat1 = +values.stat1 || 0;
const stat2 = +values.stat2 || 0;
const sum = stat1 + stat2;
console.log('starting');
let my_string = "Some Displayed Stats:\n";
my_string += ' Stat1: ' + stat1 + ';\n';
my_string += ' Stat2: ' + stat2 + ';\n';
my_string += ' Sum: ' + sum + '.';
console.log(my_string);
});
});
Code language: JavaScript (javascript)
Assuming stat1 = 10 and stat2 = 5, that produces the same output. But tracking all the quotes and the \n operators can be a bit messy. Using a template literal, you could use:
on('sheet:opened', function() {
getAttrs(['stat1', 'stat2'], function(values) {
on('sheet:opened', function() {
getAttrs(['stat1', 'stat2'], function(values) {
const stat1 = +values.stat1 || 0;
const stat2 = +values.stat2 || 0;
const my_string = `Some Displayed Stats:
Stat1: ${stat1};
Stat2: ${stat2};
Sum: ${stat1 + stat2}.`
console.log(my_string);
});
});
Code language: JavaScript (javascript)
Things to notice here:
- Since the variable is created just once, we only need to use const in place of let.
- The extra spaces at the start of each line are inserted naturally
- the sum calculation is performed in place (${stat1 + stat2}).
- Adding the punctuation at the end of each line is easy.
You can nest ${} operations inside other ${} for incredibly complex calculations, and ternary operators are a great way to perform conditional calculations. For instance, say you just want to print out stat2 if its over 10:
const my_string = `${stat2 > 10 ? `Stat2: ${stat2}` : ''}`
console.log(my_string);
Code language: JavaScript (javascript)
This is an example of nested calculations. It gets quite difficult to read these the more nesting you have, and if readability is important you can build the strings with more old-fashioned code. But template literals and ternary operators are useful skills to have in your toolbox.