- Anatomy of a Sheet Worker
- Events, and watching Attributes
- Variables – How to Name Things
- Arithmetic in Sheet Workers
- What If? in Sheet Workers
- JavaScript Objects
- Getting Loopy With JavaScript
- Logging in the Browser Console
- Strings, Arrays, and Loops
- Asynchronicity and Things to Avoid With Loops
- Changes and the eventInfo Object
- Action Buttons
- setAttrs and Saving Attributes
- Castle Falkenstein Design – Sheet Workers
- The Perils of Sheet Worker Functions
- The Script Block and Identifying Characters
- Arrays and Dropdowns
- Undefined and Other Error Values
- The Ternary Operator – The One-Line If
- Template Literals
- Functions and the Fat Arrow
- Strings in Sheet Workers
- A Sheet Worker Reprise
I have talked about Variables before, where types of data were described. The articles on Objects, Arrays, and Strings talk about specific data types and how useful they can be (especially in combination). But there is a specific category that can be thought of as Unvariables, data that isn’t actually a data type.
Undefined, and Errors
You will probably only see the undefined value when you have made an error.
on('change:character_name', function() {
getAttrs(['character'], function(values){
const name = values.character_name;
console.log({name});
});
});
Code language: JavaScript (javascript)
Let’s say you tried this. This is what you’d see in the console:

on('change:character_name', function() {
getAttrs(['character_name'], function(values){
const name = value.character_name;
console.log({name});
});
});
Code language: JavaScript (javascript)
This gives a much more worrying error:
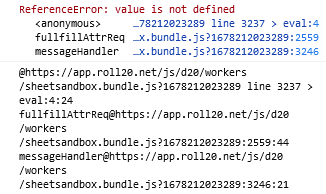
Here, value is a typo and a more serious one. There is no value object already defined (it should be values). This is a critical error – the sheet worker itself fails, and it might stop all sheet workers. But the error is a simple typo, so let’s replace it with a different typo:
on('change:character_name', function() {
getAttrs(['character_name'], function(values){
const name = values.character_nam;
console.log({name});
});
});
Code language: JavaScript (javascript)
Now we are back to that undefined error because the attribute name is not included in the values object.
What To Do Now
Undefined (and its major sisters null and NaN – not a number) is a value, but it’s not a string, number, or any other data type. You can’t do anything with it. You have to find why its undefined, and get rid of it. This is the main reason we do this:
const score = Number(values) || 0;
Code language: JavaScript (javascript)
The || 0 there makes sure the result is a number. If we try to convert a string like “Strength” to a number, it will be undefined, because “Strength” is not a number.
So you need to build your code to avoid unvariables. You can still use unvariables in expressions. This works:
if (score === undefined) {
Code language: JavaScript (javascript)
typeof
There is a function which lets you query the type of data (string, number, etc) and it will tell you if something is undefined. Generally speaking, though, it’s better to construct your code in such a way that you never see undefined.
This is where logging comes in very handy. When you are testing sheets, log liberally, find what kind of expressions produce undefined values, and change your code to avoid them.
Remember you can’t do any of the things you want to do with undefined. You can’t perform arithmetic, or combine it into longer strings. Get rid of it.